In today’s competitive app ecosystem, Android developers must focus on efficiency, security, and user experience (UX) to build successful apps. While it’s easy to get caught up in adding features, these three pillars form the foundation of a great mobile application. Here are the crucial points every Android developer should keep in mind when developing any project.
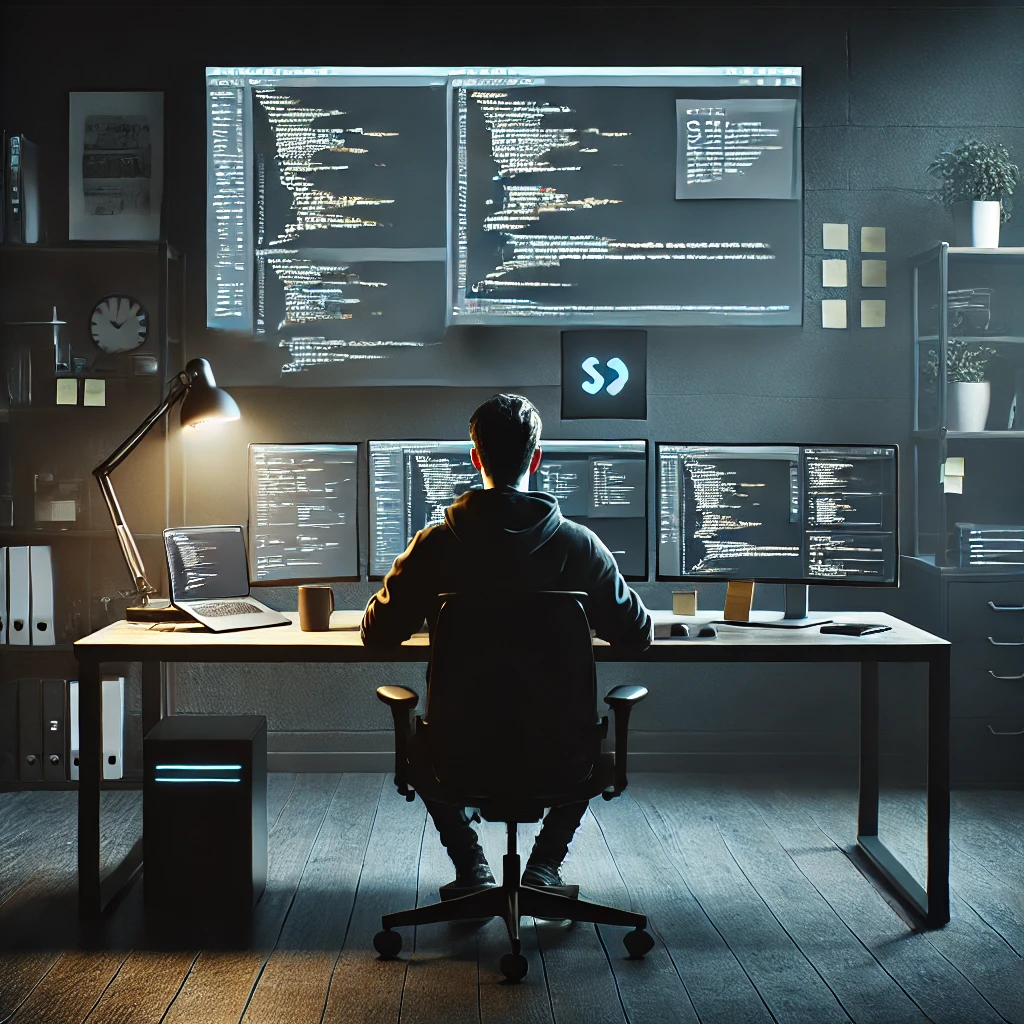
1. Efficiency: Optimizing Performance
App performance is critical. An efficient app loads quickly, consumes minimal memory, and provides a smooth user experience. Here’s how to ensure efficiency in your Android app:
a. Minimize UI Overdraw
Overdraw occurs when the same pixel is drawn multiple times in a single frame, leading to unnecessary rendering and slowdowns. Use Android Studio’s Debug GPU Overdraw tool to identify and minimize overdraw by reducing the complexity of your layouts.
b. Use Efficient Data Structures
Choose the most appropriate data structures to store and process data. For example:
- Use SparseArray instead of HashMap for integer keys, as it saves memory.
- Avoid using nested data structures that can lead to performance overhead.
c. Leverage Background Processing Wisely
Offload heavy or long-running tasks to background threads using WorkManager, AsyncTask, or Coroutines. Ensure that background tasks are handled efficiently without blocking the main UI thread, which can cause your app to freeze or lag.
d. Use View Binding or Data Binding
Both View Binding and Data Binding can help reduce the amount of boilerplate code in your app, improving performance by eliminating the need for findViewById()
. View Binding also helps reduce the chances of memory leaks, which can cause the app to crash or slow down.
e. Optimize Images and Resources
Large image files can slow down your app’s loading time and consume unnecessary memory. Use vector drawables for scalable icons and images, and compress raster images (like PNG, JPG) using WebP format, which significantly reduces image size without loss of quality.
2. Security: Protecting User Data
Security should be top-of-mind for every developer. With increasing privacy concerns and regulations like GDPR, it’s vital to secure user data and prevent vulnerabilities in your app.
a. Implement Secure Data Storage
Never store sensitive information (e.g., user credentials, API tokens) in plain text or SharedPreferences. Instead:
- Use Android Keystore for securely storing cryptographic keys.
- Encrypt files using AES encryption and ensure secure database storage with SQLCipher.
b. Use HTTPS Everywhere
All network communications should be encrypted with HTTPS to protect against man-in-the-middle attacks. Use SSL pinning to ensure that your app only communicates with trusted servers and detect if someone is trying to intercept the connection.
c. Handle Permissions Carefully
Android provides granular control over permissions. Only request the permissions your app genuinely needs, and always request them at runtime (not at install time). For example:
- Use Scoped Storage in Android 10 and above to limit access to external storage.
- Always respect user privacy and avoid collecting unnecessary data.
d. Secure API Communication
When making API calls, ensure:
- You’re using OAuth 2.0 or other secure authentication mechanisms.
- Never hard-code API keys or secrets within your app code. Use secure mechanisms like environment variables or server-side configurations.
- Validate all inputs from users to avoid injection attacks (SQL Injection, XSS).
e. Code Obfuscation
Obfuscate your code using ProGuard or R8 to prevent reverse engineering. This reduces the risk of malicious users decompiling your app and tampering with sensitive logic.
3. User Experience: Building an Intuitive and Enjoyable App
A seamless, intuitive user experience is key to retaining users and improving engagement. An app that’s hard to navigate or doesn’t meet user expectations will quickly be abandoned.
a. Optimize for Different Devices and Screen Sizes
With Android being used on a variety of devices, your app needs to be responsive. Use ConstraintLayout to create adaptive layouts that adjust gracefully to different screen sizes, and support multiple screen densities by providing appropriate image assets in the res/drawable directories (ldpi, mdpi, hdpi, xhdpi, xxhdpi, xxxhdpi).
b. Prioritize Accessibility
Make your app accessible to all users, including those with disabilities. Some tips:
- Use contentDescription for images to help visually impaired users.
- Provide keyboard navigation and focus indicators for users with motor disabilities.
- Test your app with TalkBack and other Android accessibility services.
c. Keep UI/UX Simple and Intuitive
Avoid cluttered UIs and focus on simplicity. Each screen should have a clear purpose with only essential components visible. Use:
- Material Design principles to guide the layout, colors, and animations.
- TabLayout or Navigation Drawer for easy navigation between sections.
- Provide meaningful animations for transitions and interactions, but avoid overloading the app with excessive or slow animations that can frustrate users.
d. Handle App States Efficiently
Handle app states such as loading, empty, error, and success gracefully. For example, use:
- Skeleton loading screens to improve perceived speed when fetching data from the network.
- Retry mechanisms when the network fails, and always give users feedback if something goes wrong.
e. Provide Offline Functionality
For a better user experience, make sure your app works offline or with poor connectivity. Implement local caching using Room or SQLite to store data offline and synchronize when the network is restored.
4. Testing: Ensuring a Smooth and Reliable App
Rigorous testing can help you catch bugs early and ensure your app runs smoothly in different environments.
a. Unit Testing and UI Testing
Use frameworks like JUnit for unit tests to ensure that your business logic works correctly, and Espresso for UI testing to simulate user interactions and verify that the app responds correctly.
b. Performance Testing
Use Android Profiler in Android Studio to monitor your app’s CPU, memory, and network performance. This helps identify bottlenecks and optimize your app for better performance.
c. User Testing
Before launching, test your app with real users in a beta release to gather feedback and identify potential issues. Google Play’s beta testing feature allows you to test the app with a specific group before the general release.